When you create a project with Angular CLI, it creates a demo web application for you. Learn how to replace this demo with your own code.
Prerequisites
The Short Version
- In the file src/app/app.component.html, replace all of the code with the following:
<app-header></app-header>
Now, when you request http://localhost:4200/, you will see the template of the HeaderComponent rendered, instead of the demo Angular web application.
The Details
An Angular web application is a tree of nested Components. In other words, you have one Component, the AppComponent, at the root of the tree, and it has one of more child Components within it. These child Components have their own Child Components within them, and so on. The AppComponent is defined in the file src/app/app.component.ts and its template is in the file src/app/app.component.html.
By default, src/app/app.component.html will contain code for a demo web application. It will be sandwiched between 2 comments that say something to the effect:
“The content below is a placeholder and can be replaced. Delete the template below to get started with your project!”
src/app/app.component.html:
<!-- * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * -->
<!-- * * * * * * * * * * * The content below * * * * * * * * * * * -->
<!-- * * * * * * * * * * is only a placeholder * * * * * * * * * * -->
<!-- * * * * * * * * * * and can be replaced. * * * * * * * * * * * -->
<!-- * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * -->
<!-- * * * * * * * * * Delete the template below * * * * * * * * * * -->
<!-- * * * * * * * to get started with your project! * * * * * * * * -->
<!-- * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * -->
...
<!-- * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * -->
<!-- * * * * * * * * * * * The content above * * * * * * * * * * * -->
<!-- * * * * * * * * * * is only a placeholder * * * * * * * * * * -->
<!-- * * * * * * * * * * and can be replaced. * * * * * * * * * * * -->
<!-- * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * -->
<!-- * * * * * * * * * * End of Placeholder * * * * * * * * * * * -->
<!-- * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * -->
<router-outlet></router-outlet>
1. Since it is just demo code, we delete everything in src/app/app.component.html.
2. We make the HeaderComponent a child of AppComponent by including the Directive <app-header></app-header> in AppComponent’s template:
src/app/app.component.html:
<app-header></app-header>
That <app-header> Directive makes HeaderComponent a child of AppComponent. AppComponent will now render HeaderComponent and nothing else, since it has nothing else in its template. Once you make this update, Angular CLI re-compiles the code automatically. If you request http://localhost:4200/ now, you will see this:
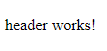
That “header works” message is the HeaderComponent rendering its template. When Angular CLI created the HeaderComponent, it put some default code in the Component’s template file, src/app/components/header/header.component.html.
src/app/components/header/header.component.html:
<p>header works!</p>
All this default code does is print out the message “header works“.
At this point, you may be wondering: Why do we reference a Component named HeaderComponent by using the Directive <app-header>? The answer: when Angular CLI generates a Component, it also creates a default Selector that has this pattern: app-[component’s name]. app is the default prefix for Angular Component Selectors.
The Selector is set in the Component’s Typescript file. For the case of HeaderComponent, it is in src/app/components/header/header.component.ts:
src/app/components/header/header.component.ts:
@Component({
...
selector: 'app-header',
...
})
Next
Now that we can at least see the HeaderComponent in our web application, we can make it do something more useful than print “header works”. We’ll learn how to do that in the next article.
Versions:
- Angular CLI: 9.1.4
- Angular: 9.1.3